Chapter 2 - The Python shell, the print statement, arithmetic, and types¶
- In the last unit we brought up the idea that using a computer to solve a problem really has two aspects:
- figuring out what the steps are in solving the problem, and then
- writing them down in such a way that a computer can interpret and carry them out.
Last time, as an example of the first aspect, we used the problem of finding the largest number in a list. Remember that we came up with a strategy for solving the problem, and wrote down the steps of the strategy as a sequence of instructions to follow. We also represented the same strategy as a picture called a flowchart. We also made the observation that human beings are really good at solving problems – so good, in fact, that it is sometimes hard for us to slow down and analyze how we’re solving the problem.
Today we want to look at the second aspect, and start learning how to write down the steps of a problem-solving strategy so that a computer can execute them. It turns out that human beings are also really, really good at language and communication. You can say all kinds of completely ambiguous things, you can use double meanings, puns, sarcasm, and allusions, and other people will usually know what you’re talking about. For example,
“Hey, bring me that thing on the table.”
“Life is like a box of chocolates.”
“The spirit is willing, but the flesh is weak.”
“Your teeth are like stars; they come out at night.”
You kin mispell woords and leave out punctuation You, can, put, in, too, many, commas, and people will still be able to read your writing.
But when you’re talking to a computer, it is just the opposite. With a computer you have to use a programming language with very precise structure. You have to get every detail of the grammar and punctuation and spelling just right, and you can’t have any ambiguity at all.
Like most computer languages, Python has the following ingredients:
keywords (such as the print keyword used below)
operators (such as +, *, <, etc .)
literal values (such as 42, 3.14, “Hello”)
identifiers (such as variables and function names)
syntax rules (grammar and punctuation)
Statements you write in Python are not directly executed by your computer’s hardware, rather, they are executed by an application called the Python interpreter. One nice thing about the interpreter is that it comes with an interactive shell, where you can easily experiment with the effects of Python statements, and the values of expressions. There are many ways of starting a Python shell. We will suggest some in the lab exercises.
Here in the text, we have an even simpler way to run short snippets of code. The little window below is called an “activecode” window. We can type and edit the Python code in the box, and click the Run button to have the interpreter execute the statements.
Try clicking the Run button below. You should see the output (47) appear on the right or below. Then, edit the text so that it says something else, like print(42 + 8)
, and try clicking the Run button again.
For example:
Let’s figure out what’s going on here.
- The whole thing,
print(42 + 5)
, is a statement. A statement is an instruction to do something. In this case, the print statement is telling the interpreter that it should display something on the screen. - 42 + 5 is an expression. An expression represents a value. In this case, the value is the number 47. Notice that 42 + 5 itself has been formed by composing two simpler expressions, 42 and 5, which are literal values.
- print is a built-in Python function
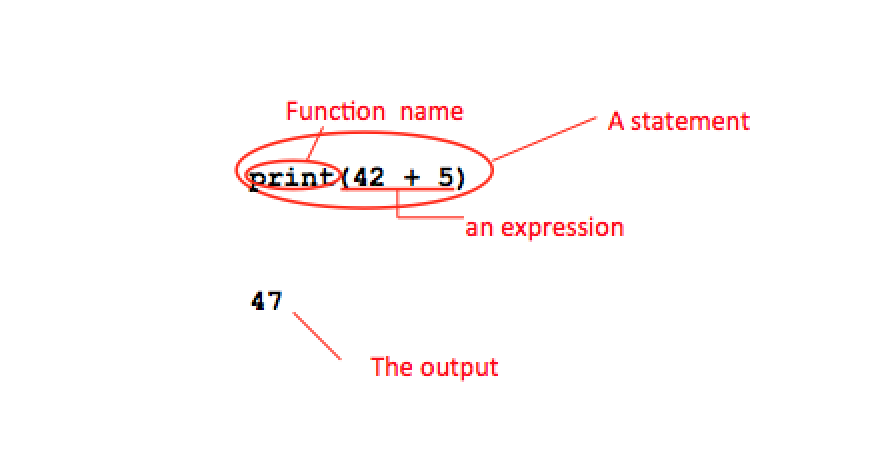
We could also display a string of text, surrounded by double or single quotes.
One thing that becomes important to us very quickly is that every value has a type. What does that mean? Well, here’s the basic idea: a computer is nothing but a whole bunch of tiny electrical switches. A switch can be “on” or “off”, and we often think of “on” as the number 1 and “off” as the number 0. Somehow those ones and zeros have to be interpreted as meaningful values like numbers and text. In order to know how it should interpret a given bunch of ones and zeros, the system needs to know what kind of value it is supposed to be - a number, text, a part of a picture, or some other type of value.
We can find out the type of a value using a built-in function called type:
Types:
Type ‘int’ means “integer” (positive and negative whole numbers)
Type ‘str’ means “string of text”
Type ‘float’ means “floating-point number”, that is, numbers with a decimal point
What’s the difference between int and float? Aren’t they both just numbers? Computers have to distinguish between whole numbers (the type int in Python) and numbers with a decimal or fractional part (the type float in Python). For numeric literals, the interpreter normally deduces the type to be float if you enter the value with a decimal point.
What about:
Well, anything with quotes around it is just a string of text. This text happens to consist of some digits and a plus sign which could be interpreted as the value 47. But since we put it in quotes, all the interpreter sees is that it is a literal string of six characters.
Now, not everything we type is going to work. Try running this:
This is called a syntax error, meaning that we didn’t follow the exact grammar for Python expressions. You’ll get a similar error if you misspell a word or use the incorrect case (as in most programming languages, everything in Python is case-sensitive). It’s a good idea to try making errors on purpose to see what happens. Then, when you make errors by accident, you’ll have some idea what’s going on.
For example, what if we forget to type the ending quotation marks?
What if we misspell the print keyword?
What if we accidentally capitalize the keyword?
As you can see, the error messages don’t always do a very good job of explaining what’s going wrong! When we start running Python scripts (as opposed to these activecode windows) the error messages may be slightly different (and more informative). Here is a useful tip to remember: when you get an error message in the shell, start reading it at the bottom. Usually the last line in the error message gives you the best clue. Unfortunately, the interpreter doesn’t always “know” what went wrong, it just reports the first place it got stuck trying to interpret what you typed.
Expressions can be composed from other expressions using the arithmetic operators. These are the ones you are familiar with, except that a star is used for multiplication instead of a dot or “x”.
(+) add
(-) subtract
(*) multiply
(/) divide
You can also use parentheses to change the order of operations:
There are two more arithmetic operators.
(//) integer division
(%) modulus
Try this
This corresponds to the kind of division you used to do in grade school:
“25 divided by 10 is 2, with 5 left over”.
So that’s where we get the answer 2. The percent sign “%” is called the modulus operator. We read this as “25 mod 10”. It just means
“the remainder when 25 is divided by 10”.
If one or both of the numbers has type float, the interpreter will perform a floating-point division even if you use the //
operator.

These activecode windows, as well as the shell, re useful for experimentation, but if you want to do anything useful involving more than a couple of statements, you don’t want to have to retype them in the shell every time. Fortunately, we can type up Python statements and save them in a file to use later. Such a file is called a script or program.
The statements in the script aren’t executed until we tell the Python interpreter to run the script. When we run this script, we see the output:
Notice that first line starting with the pound sign “#” doesn’t seem to be having any effect when the script runs. It is called a comment. Comments are ignored by the interpreter but are useful for us, because they can help explain what the script (or part of the script) is for.
In the shell, you can just type an expression like 2 + 3, and the interpreter will evaluate it and display the value immediately. That’s just the way the shell works. But if you think about it, 2 + 3 really isn’t an instruction to “do” something with the value 5, it just “is” the value 5. So in a script, writing an expression by itself has no effect. For example, when we run the following script, the output is just:
We always try to distinguish between expressions and statements in Python.
- A statement is an instruction to do something. So far, the only kind of statement we know about is the print statement.
- An expression represents a value, such as 2 + 3 or “Hello”.
Try adding an extra space before the print keyword and see what happens. Notice the error message. This reveals one distinctive feature about Python: indentation matters. We will see later how indentation is conveniently used to show the structure of more complicated scripts.
There is one other bit of jargon to get used to: programmers refer to anything written in a programming language as “code.”