Lab 8 - Indexing and Strings¶
Part 1: Indices and brackets¶
We have seen a lot of examples of loops that iterate over every element of a sequence. For example, here is a function that finds the sum of the elements in a list of numbers.
We have also seen that we can directly get an element from a list using its index and the “bracket” notation. The first element of a list has index 0, and the last element’s index is the length of the list, minus 1.
For example, we used this code to select a random color from a list of possible colors. (Try running it multiple times.)
We can use the index for iterating over a list too. The first element always has index 0, and the last element is the length minus 1. The sum
function above could be written like this:
Step through this loop carefully in codelens. Watch how the loop variable index takes on values 0, 1, 2, 3 from the range, and then num = a_list[index]
gets the actual value in the list.
The second version of sum
is clearly more complicated the first one. Why would anyone want to do this??
The answer is that using an index gives us a lot more flexibility in accessing the values in the list. Now we can start in the middle of a list, compare multiple elements, iterate in reverse, and even make modifications to it within the loop.
In class we saw this example, that determines whether a string has a double letter. The index is useful because we need to examine two characters of the string at a time.
Checkpoint 1¶
- Write a function that given a list, prints the elements in reverse.
2. Write a function that given a list of numbers, determines whether the list is in ascending order.
Part 2: Strings and substrings¶
The box below contains a summary of the most common string methods.
You can also see more of them in the Python Library Reference:
http://docs.python.org/library/stdtypes.html#string-methods
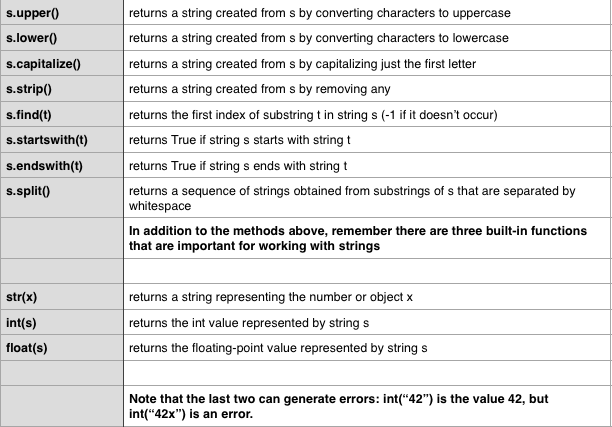
In addition to the string methods above, the keyword in
is useful with strings. (The keyword in
is also used when we write for-loops, but this is a different usage.) For strings s
and t
, the expression
t in s
has value True if string t
is a substring of string s
.
Another example would be a function that determines whether or not a given character is a vowel:
Sometimes it’s not enough just to know whether one string is a substring of another; we need to know the index where it occurs. For that we use the find
method.
Another useful method for taking apart strings is split
. The result of calling s.split()
is a list of strings, each of which is a “word” within the original string s
. (Here a “word” is just any group of characters separated by spaces or tabs.)
Checkpoint 2¶
- Write a function that, given a string, returns a new string obtained by exchanging the first and last letter. (For example, given “boogers” the function returns “soogerb”.)
- Write a function that, given a time string such as “1:28” or “10:30”, returns the number of minutes represented. (For example, given “1:25”, the function returns the number 85.)
Part 3: Strings and indices¶
Since the characters in a string can be indexed like a sequence, we can iterate over them using a for loop directly or using an index. For example, we could count the number of times the letter ‘p’ occurs in a string.
We can also use the index if we needed it. For example, here is a function that finds the index of the first ‘p’. Similar to the find
method of Python strings, it returns -1 if a ‘p’ is not found.
Checkpoint 3¶
- Write a function that finds the index of the first vowel (‘a’, ‘e’, ‘i’, ‘o’, or ‘u’) in a string.
- Write a function that converts a word to piglatin according to these rules:
if the word starts with a vowel, add “way” to the end
otherwise, all characters before the first vowel are moved to the end, and then “ay” is added.
E.g. “banana” becomes “ananabay”, “grumpy” becomes “umpygray”, and “apple” becomes “appleway”.
- Write a function that takes a sentence of text (without punctuation), and prints it out in piglatin. (Hint: Start with the
split()
method and iterate over the words, as in the example above usingsplit()
.)
Another useful trick: if you want to call print()
but you don’t want to go to the next line, you can add the option end=""
to the print function, like this: