Lab 3 - Conditionals¶
Conditional statements and boolean expressions (Python expressions with value True
or False
) were introduced in Eels, chapter 5. Refer back to that chapter if the questions below seem unfamiliar to you.
Part 1: Conditions¶
Checkpoint 1¶
Suppose you have integer variables x
, y
, and a string variable name
defined as shown in the activecode window below. Write a boolean expression capturing the meaning of each statement below, and print the value of the expression. The first one is done as an example.
- x is at least 5
- x is equal to 6
- y is positive
name
has at most 10 characters- y is divisible by 3
- y is not divisible by 3
- x is less than or equal to the square root of y
- the sum of x and y is 42
- the square of y is at most 100
name
is “Steve”
Part 2: Conditional statements¶
Problem 1
Burritos-R-Us sells delicious burritos in their Ames, Iowa store. Burritos are $6.00 each, plus a delivery charge of $5.00 per order. However, they offer free delivery for orders within zip code 50011.
- (pencil and paper) How much is an order of 5 burritos in zip 50011? How much is an order of 10 burritos in zip 50013?
- In Wing 101, write an interactive Python script that reads in the number of burritos and the zip code, and prints the total price for the order. Verify that your program gives the same answers as your pencil-and-paper calculations.
Problem 2
Printed T-shirts are $10.00 each, but if you order fewer than 30 shirts you have to pay a $50 setup charge. On top of that, Minnesota residents need to pay 5% sales tax.
- (pencil and paper) How much is an order of 20 shirts for a Minnesota resident?
- In Wing 101, write an interactive Python script that prompts the user to enter the number of shirts, and then prompts the user to enter “y” or “n” (Minnesota resident or not), and then prints out the total cost of the order. Verify that your program gives the same answers as your pencil-and-paper calculations.
Checkpoint 2¶
Show the TA your hand calculations and your completed Python programs for problems 1 and 2 above.
Part 3: Nested conditionals¶
Here is a simple variation on Problem 1 above. Suppose the burrito place instead has the policy that delivery is free for orders in zip 50011 only if the order is for 6 or more burritos; otherwise delivery is $3.00. (For orders outside zip 50011, delivery is still $5.00.)
First, take a moment and recompute the answers to the questions from part a: How much is an order of 5 burritos in zip 50011? How much is an order of 10 burritos in zip 50013?
Notice that in order to find the total, you have to answer two questions: is the order for zip code 50011, and if so, is it for 6 or more burritos?
We can picture this sequence of decisions as a flowchart. Here assume that num
and zip
have been previously defined.
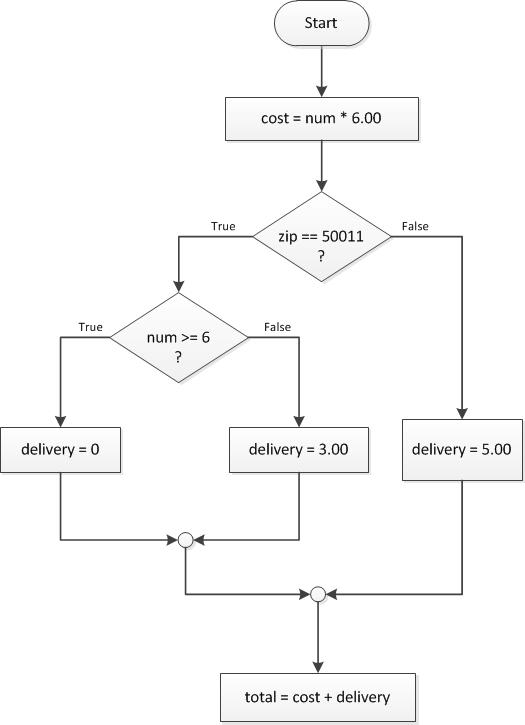
In order to write the code, we have to have a conditional statement within a conditional statement. Since the first if-block is already indented, we have to indent to another level to create a conditional statement within it.
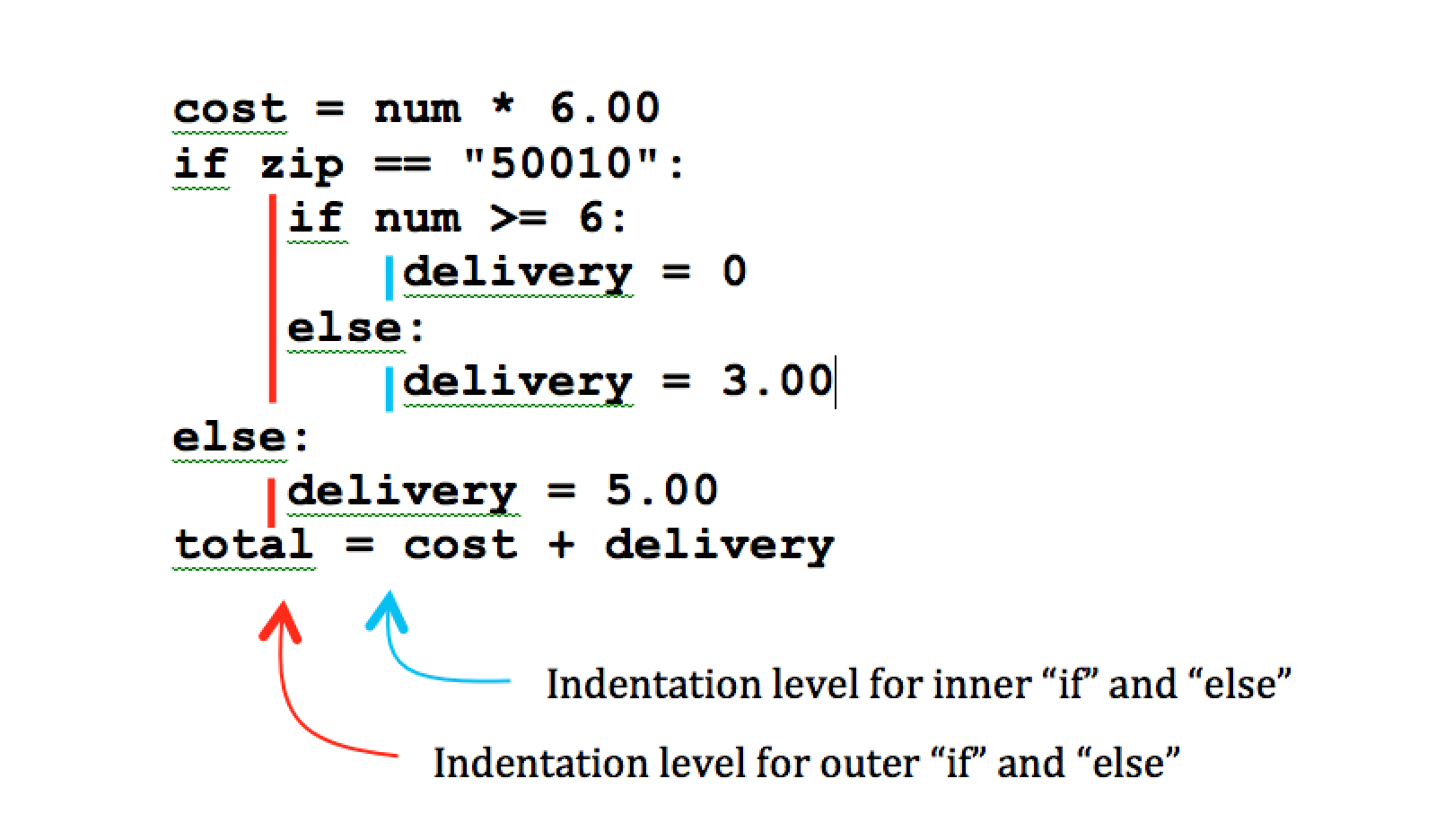
(The input
and print
statements are omitted above.)
Checkpoint 3¶
Suppose you thought about the burrito problem differently. What if you first determine whether the order is for 6 or more burritos, and then ask whether its for zip 50011. Then the logic would correspond to the flowchart below.
Write a Python program for computing the order total that corresponds to this flowchart.
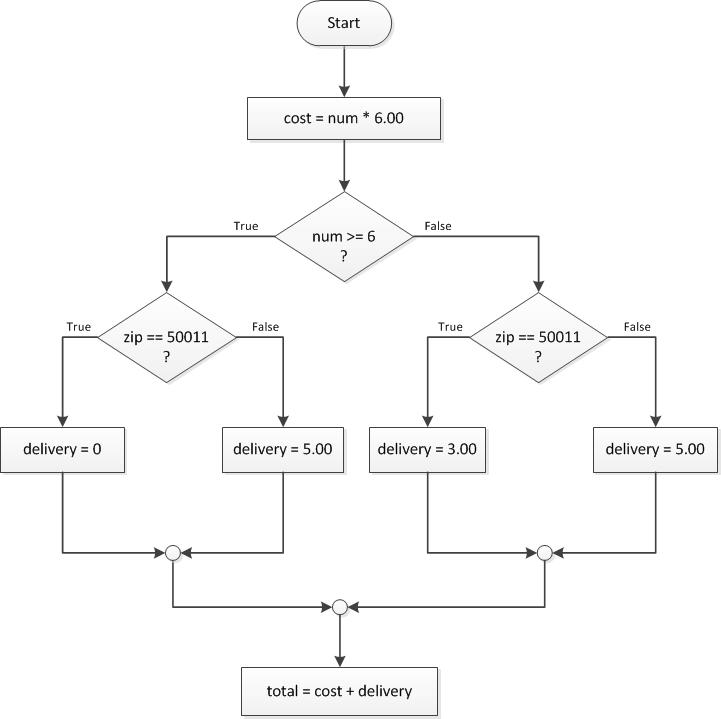
Part 4: Creating a flowchart from a problem statement¶
Normally, labor laws require that employees who are paid an hourly wage have to be paid extra whenever they work more than 40 hours per week. The extra is usually 11⁄2 times the regular hourly wage for all hours above 40. However, an exception is made for employees with “exempt” status, which is meant for positions such as managers and supervisors. They don’t get paid any extra for overtime.
Compute the total wages for the examples below using a pencil and paper:
- 20 hours, rate $10.00 per hour, status “non-exempt”
- 20 hours, rate $10.00 per hour, status “exempt”
- 44 hours, rate $10.00 per hour, status “non-exempt”
- 44 hours, rate $10.00 per hour, status “exempt”
(Results: 200, 200, 460, 440)
Next:
- Sketch a flowchart representing the calculation.
- Write a Python program that takes three input values
hours
,hourly rate
andstatus
and prints total wages.
Checkpoint 4¶
Show your flowchart and demonstrate the Python program to the TA.